This is lesson four of a 10 ten-lesson course, Simple CodeIgniter App, which walks you through the creation of your first PHP web application using the CodeIgniter framework.
Before we jump into any writing code for our web app, it’s important discuss some of the basic concepts of programming in general.
And since we’ll be using it to write most of our app’s code, we’re going to use PHP to help demonstrate all of these concepts.
PHP is great to use as an example as it contains almost all of the common abilities shared nearly universally between the great majority of programming languages in existence.
A simple example
PHP lets you easily perform common tasks, such as mathematical operations, date and time calculations, and much more.
At its simplest, a snippet—or small piece of code—of PHP to calculate the result of 8 times 4 would look like this:
<?php
8*4
?>
with the *
representing the multiplication operator (which is the case in almost all programming languages, by the way).
Remember, as mentioned in a previous lesson, all blocks of PHP code must be surrounded by opening, <?php
, and closing, ?>
, PHP tags.
With that said, any number of lines of PHP code can exist within those opening and closing tags. So, for example, (as you’ll see later on) if we have an entire file filled with only PHP code, we only need one opening tag at the very beginning of the file and one ending tag at the very end.
Now back to our code. Just running an isolated calculation like 8 * 4
doesn’t do us a whole lot of good. Thankfully, PHP allows us to store pieces of information in variables.
Variables help us store information
Variables are named placeholders that allow us to reuse information throughout our code, simply by referring to them by name.
So, if we wanted to to store the result of our previous calculation (say, to use it again at a later point in time), we could do the following to store it in a variable named $result
:
<?php
$result = 8 * 4;
?>
This effectively says to our code: “Compute the result of 8 times 4 (the right side of the equals sign) and store it (as designated by the equals sign, itself) in a variable named $result
.”
Naming variables in PHP
Note that when defining a variable in PHP, its name must be preceded with the <em>$</em>
symbol. Additionally, all variable names must be just one word, without spaces, containing only letters, numbers, and underscores, _
. Additionally, the name must start with a letter.
So, for example, <em>$result</em>
, <em>$this_result_2</em>
, and <em>$this_3rd_result</em>
are all valid variable names in PHP.
The result of our calculation, 32
, is now stored in the variable $result
. We can then, as mentioned, use it again within another piece of code, like so:
<?php
// $another_result will hold the value 42
$result = 8 * 4;
$another_result = $result + 10;
?>
Using comments to explain your code
There are a few things to note in the code above. First, you can see the use of a comment in the first line:
// $another_result will hold the value 42
Comments can be added as a way to describe a piece of code and are created by preceding a line with a double forward slash, //
, as shown. Note that comments are not considered as actual lines of code, and they won’t ever have any effect on the code, itself. They’re simply there to make it clear— to someone else who may be reading your code (or to remind yourself)—as to what the code should be doing.
If we wanted that same comment to span multiple lines, we could begin it with /*
and end it with */
, like so:
<?php
/*
* $another_result
* will hold the
* value 42
*/
$result = 8 * 4;
$another_result = $result + 10;
?>
which is known as a block comment.
And finally, every operational line of code—every line directly responsible for some functionality, such as defining a variable or performing a calculation— must end with a semicolon, ;
. The semicolon essentially says to the code, “Okay, this operation is now complete. Move on to the next, please!”
Printing things out
Very frequently when building our app, we’ll be using PHP to print out pieces of information in amongst HTML code.
To accomplish that, we’ll use PHP’s echo
function.
For example, to print out a simple piece of text, we might write the following code:
<?php
echo 'The sky is blue?’;
?>
which, when used within a webpage’s code, would simply display:
The sky is blue?
within a browser.
Note that you can also print out the values of variables:
<?php
$our_sentence = 'My cat is round.’;
echo $our_sentence;
?>
which would display:
My cat is round.
Packaging code functionality using functions
Frequently, when coding, you’ll write a group of code lines all designed to work together to perform a specific task.
In PHP (as well as in all other programming languages), we’re given a very convenient way to organize such pieces of code: by defining functions.
Let’s take the following (simple) example of a PHP function that can be used to print out someone’s name:
<?php
function print_my_name($name) {
echo 'My name is ' . $name . '.';
}
?>
Notice that the above function accepts one parameter: $name
. Parameters are used to allow us to pass required information into a function. In this case, our function does not work properly unless we pass it a name.
We can call our function (i.e. execute it) by using its name and passing in the necessary parameter value(s), like so:
<?php
print_my_name('Alex');
?>
The above code would print out:
My name is Alex.
by joining three strings: "My name is”
; the value of our $name
variable that was passed in ("Alex”
in the example above); and the string containing just a period, ".”
, to end the sentence.
You also just saw the first example usage of string concatenation—combining multiple strings into one. In PHP, this is done using the concatenation operator, .
, which, as you can see, is just a dot/period.
Operators allow us to perform various operations, such as joining two strings with the dot, .
, operator or comparing two numbers with greater than, >
, or less than, <
operators.
To return or not to return: two examples
Functions have the ability to send information back to the location in the code from which they were originally called. When they do this, it’s referred to as returning.
All functions can either: a) return some sort of information; or b) not return anything. Both are used quite frequently—whether or not a function returns any information is totally dependent on the situation it’s handling.
Let’s take two examples to describe when it may, and may not, be appropriate for a function to return information.
In our first example, we have a function that’s used to print out the brand, model, and color of a car.
The function, as well as a piece of code that executes it, may look something like this:
<?php
function print_car_info($brand, $model, $color) {
echo 'I drive a ' . $color . ' ' . $brand . ' ' . $model . '.'.
}
// The statement below should print "I drive a red Dodge Stratus."
print_car_info('Dodge', 'Stratus', 'red');
?>
In this case, there’s no information that needs to be returned for further use; we’re simply printing out a string.
Now let’s take a second example where we do need our function to return some information.
Let’s say we have four numbers and we want to: multiply the first two together; multiply the last two together; and then get the sum of those two results.
The function and additional code to accomplish that may look like this:
<?php
function multiply_numbers($number_one, $number_two) {
$result = $number_one * $number_two;
return $result;
}
// The four initial numbers:
$first_number = 6;$second_number = 16;$third_number = 19;$fourth_number = 87;
// This call to multiple_numbers should produce 96,
// which will be stored in $first_times_second
$first_times_second = multiply_numbers($first_number, $second_number);
// This call to multiple_numbers should produce 1653,
// which will be stored in $third_times_fourth
$third_times_fourth = multiply_numbers($third_number, $fourth_number);
// This addition operation should produce 1749,
// which will be stored in $answer
$answer = $first_times_second + $third_times_fourth;
?>
In the example above, you can see that our function does something rather simple: multiplies two numbers together. What’s important is the fact that it returns that result so that it can be stored in a variable and used at a later time.
We begin with our four initial numbers. We then pass the first two numbers into our multiply_numbers
function, returning that $result
, and storing that value in our $first_times_second
variable.
Without that last return
statement in our function, we would never have access to the resulting value, as it would never be sent back to the place in our code where we originally called the function. But with the return
statement, we get the resulting value.
We can then do the same thing for our third and fourth numbers, and finally, retrieve our $answer
value by summing those two results.
Variable and function locations and scope
You may have noticed in the previous code example that our function was defined at the top and then called afterwards:
<?php
function multiply_numbers($number_one, $number_two) {
$result = $number_one * $number_two;
return $result;
}
.
.
.
$first_times_second = multiply_numbers($first_number, $second_number);
?>
That’s generally good practice—defining functions before they’re called—but it’s not entirely necessary. When a PHP file is accessed, all of its functions are initialized before any of the other lines of code are executed. So as long as a function is defined in the same file, it can be called from anywhere within that file.
For example, we could have called our multiply_numbers
function before it was defined, and we still would’ve gotten the desired results:
<?php
$first_times_second = multiply_numbers($first_number, $second_number);
.
.
.
function multiply_numbers($number_one, $number_two) {
$result = $number_one * $number_two;
return $result;
}
?>
This is also a good time to address a concept known as scope. The scope of a variable refers to the domain within which it can be referenced.
In the case of functions, their code is entirely self-contained, so any variables initialized and contained within a function itself are only available within that function. Let’s look at an example:
<?php
function example_function() {
$function_var = 'hello';
return $function_var;
}
// This line will return an error, as $function_var
// only exists within example_function
echo $function_var;
?>
In the code above, we have a function, example_function
, and within that function, we’ve define a variable, $function_var
. The function’s code is completely valid. We define a variable and then return it.
But below that, you see another statement, outside of the function, trying to echo
the $function_var
variable that was created inside of example_function
. That line of code is invalid, as the $function_var
does not exist outside of example_function
.
And that’s a perfect demonstration of why we require the use of return
statements— to make use of values contained within a function outside of it.
Using arrays to store multiple items at once
In programming, one of the most commonly used data structures (i.e. a particular way of storing or representing data) is the array.
An array is used to store a group of associated values, together. And as you’ll see as we progress through building our app, they’ll become extremely useful in all sorts of different situations.
Let’s look at an example to see how arrays function. In this example, we’ll be using an array to store a group of numbers.
Adding items to an array
Let’s start by creating an empty array:
<?php
$our_numbers = array();
?>
Now, let’s add a few numbers to it:
<?php
$our_numbers[] = 1;
$our_numbers[] = 2;
$our_numbers[] = 3;
?>
Great! Now our array contains three numbers: 1, 2, and 3. In PHP, you’ll notice that the syntax for initializing a new, empty array is:
$array_name = array();
and the syntax for adding an item to an array is:
$array_name[] = <value-to-add>;
where $array_name
is the name of the array variable, immediately followed by open- and closed-brackets, []
, followed by the equals sign and the value you wish to add to the array.
Creating an array with items in it right off the bat
Above, we created our array first and then proceeded to add values to it after the fact. In PHP, you can also create an array initially that already includes values. To create our same numbers array in this manner, we could write:
<?php
$our_numbers = array(1, 2, 3);
?>
The values you wish to include in the array are simply included within the array function’s parentheses, separated by commas, as seen above. And we’re not restricted to a certain number of values; we could create an array with 50 or even 500 initial values, if desired.
Arrays are like dictionaries
In PHP, arrays act, essentially, like dictionaries: you add an item to the array and assign it an associated key, so that you look the item up by that key later on. In an actual dictionary, you have words and their associated definitions; in arrays, you have keys and their associated items, or values.
So, for example, if you were to add a value to an array with the key 'dog’
, you would later be able to look up/retrieve that value using the 'dog’
key:
<?php
$dictionary = array();
$dictionary['dog'] = 'A canine companion';
echo $dictionary['dog']; // will print out 'A canine companion’
?>
Note that this is very similar to a dictionary: you know a word (i.e. the key), say “computer”, and you can look up its definition (i.e. the value) by locating it in the dictionary, as depicted below.
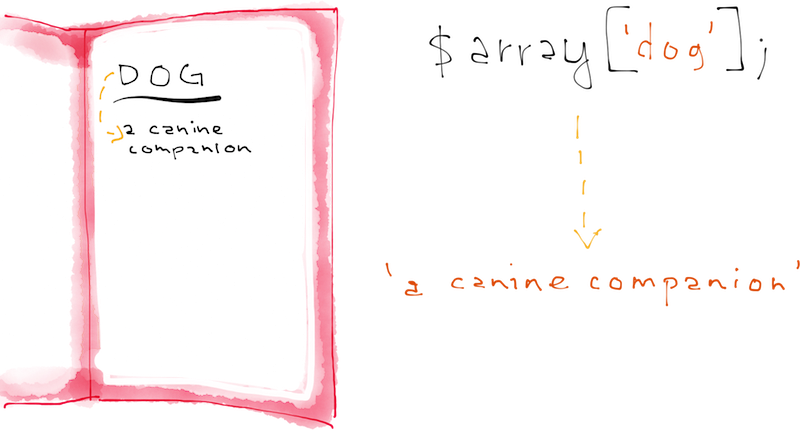
Retrieving array values where keys weren’t specified
On the other hand, if you created an array without explicitly designating keys:
<?php
$our_numbers = array();
$our_numbers[] = 1;
$our_numbers[] = 2;
$our_numbers[] = 3;
?>
auto-incrementing integers, starting at 0, are automatically assigned as keys. So, for example, to retrieve to first value in the array, you would use:
<?php
// $first_value will contain 1
$first_value = $our_numbers[0];
?>
And to retrieve the third value, you could use:
<?php
// $third_value will contain 3
$third_value = $our_numbers[2];
?>
Programming indexes start at 0, not 1
In almost all areas of programming, when you’re talking about the index, or location within a larger group, of an item, the count starts at 0, not 1, as depicted:
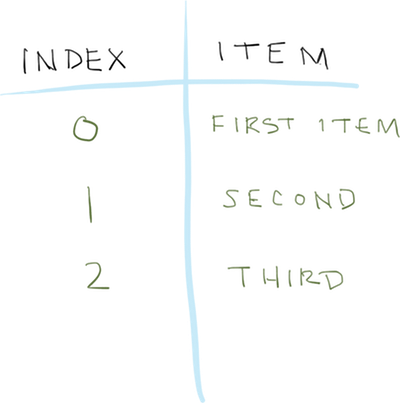
Using loops to perform multiple operations
Frequently—in all kinds of programming, including web development—we need to either: 1) perform a very similar operation, many times in a row; or 2) iterate, or cycle, through a collection of items—such as an array—and perform a certain function on each item.
To accomplish that, we can use what’s known in programming as a loop.
foreach
loop example
Let’s take a look at a quick example. Say we have an array of numbers—such as the $our_numbers
array we created above—and we want to find the square root of each of them. In programming, we can very conveniently use a loop to help us do just that:
<?php
// Our initial array of numbers
$our_numbers = array(1, 2, 3);
// A new array to store our numbers' square root values
$our_numbers_roots = array();
// Loop through each of our numbers in order to
// compute all of their square roots
foreach ($our_numbers as $num) {
// Compute the current number's square root
// and add it to our square roots array
$num_root = sqrt($num);
$our_numbers_roots[] = $num_root;
}
?>
As you see above, we start off with our initial array of numbers, $our_numbers
, as well as a new, empty array, $our_numbers_roots
, which will store the square root values we’ll be computing.
We then utilize a foreach
loop to cycle through all of our numbers.
foreach
loops allow us to take an array of items and go through each one, starting with the first one and automatically ending with the last one.
As seen above, the syntax for a foreach
loop is as follows:
<?php
foreach ($array_name as $variable_representing_current_item) {
// Any necessary actions
}
?>
The structure of a foreach loop
The parentheses immediately following the foreach
contain the following three
things:
- the name of the array that we wish to loop through;
- followed by the keyword as;
- and a variable that represents the current item, which can be named anything of our choosing (as you see in the example above, we used the variable named
$num
to represent each individual number as we looped through them).
The process of looping through an array’s items using a foreach
loop is demonstrated in the figure below.
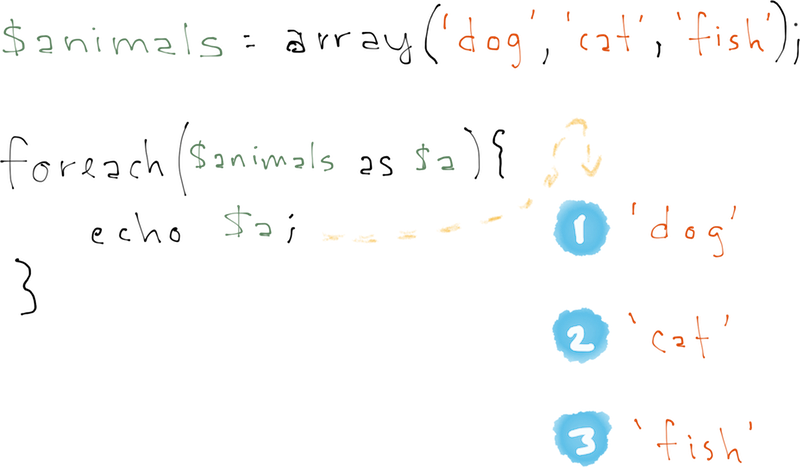
for
loops
In PHP (and again, nearly all other programming languages) we also have what’s known as a for
loop, which functions slightly differently than our foreach
loop.
for
loops take on the format:
<?php
for ([initial variable]; [condition]; [to-do after each loop]) {
[code to execute]
}
?>
So, for example, if we wanted to generate an array of numbers to represent the different months in the year, we could do the following:
<?php
$months = array();
for ($i = 1; $i <=12; $i++) {
$months[] = $i;
}
?>
That translates to the following:
- “Start with a variable,
$i
, equal to1
; - as long as
$i
is less than or equal to12
, continue looping; - after each loop, increment
$i
by one ($i++
, which is the same exact thing as$i = $i + 1
)”.
That means that our loop will run the first time with $i
equal to 1. After that, $i
will be incremented by 1
—updating its value to 2
—which is still less than 12
, so the loop will run again.
And so on, all the way through $i
being equal to 12
.
After that, $i
will be incremented and set to 13
, and our condition, $i <= 12
will return false
, thus exiting the loop.
You can see how this loop works perfectly for generating a list of numbers in order.
Recap
You now have a solid background of some of the most important, fundamental concepts in all of programming: variables, functions, arrays, and loops.
And we’ll continue to use all of these concepts throughout the remainder of the course as we build our web app, so feel free to refer back to this lesson as often as you’d like.
Now it’s time to put your new knowledge into action.
Week 4 Task
Write some PHP code of your own that utilizes variables, functions, arrays, and loops.
Now that you have an understanding of these four coding concepts and have seen multiple examples of each, it’s time to write some code of your own that puts them to work. After working through the previous lessons, you should already have your computer set up for writing PHP code.
1. Create a new file
Start by creating a new test.php
file directly within your web root directory (C:wampwww
for Windows; /Application/MAMP/htdocs
for Mac), and open it up within Sublime Text.
2. Complete the following exercise (or feel free to come up with one on your own!)
- Create an array that contains a few names—maybe one that contains your name as well as a few of your friends’ names.
- Write a function that accepts two parameters—a person’s name and the number they are within the array (i.e. the second person’s number would be 2)—and returns a message in the format: “[name] is person #[number].” (So an example message may be “Alex is person #1.”)
- Use a loop to iterate through your array, calling your function to print out all of these messages. Remember that you’ll have to somehow keep track of what number person you’re on. (Hint: you can use a counter to do this.)
When you’re finished, leave a comment below with your working code. (Make sure to test it by accessing your new file it within your browser!)
BONUS:
- Have your array store both names and the person’s relationship to you. So, if you’re adding your sister Jane, her “name” would be “Jane” and her relationship (to you) would be “sister”. (Hint: You’ll need to use a multidimensional array in order to accomplish this.)
- Then alter your function to accept three parameters—name, number, and relationship— and return a message in the format: “[name] is person #[number] and is my [relationship].” (e.g. “Jane is person #1 and is my sister.”)
And as always, feel free to send me an email or leave a comment with any questions you have or reach out if you get stuck. I’m always here to help!